This guide to Arduino memory is intended to help anyone understand how much memory an Arduino has, what that memory is used for, and what happens when more memory is needed. I’ve also included the results of an experiment I ran to find out what happens when an Arduino runs out of memory.
An Arduino typically has between 2 and 96 kilobytes of random access memory for storing variables and between 32 and 512 kilobytes of program memory for storing sketches. Many Arduino also have around 1 kilobyte of EEPROM which can be used to store variables that must be saved even if the Arduino loses power.
Model | SRAM (KB) | EEPROM (KB) | Program (KB) |
---|---|---|---|
UNO Rev 3 | 2 | 1 | 32 |
Mega 2560 Rev 3 | 8 | 4 | 256 |
Nano | 2 | 1 | 32 |
Due | 96 | 0 | 512 |
Leonardo | 2.5 | 1 | 32 |
Micro | 2.5 | 1 | 32 |
UNO WiFi Rev 2 | 6 | 0.25 | 48 |
MKR WiFi 1010 | 32 | 0 | 256 |
Overall, the Arduino Due has the most memory with 96KB of SRAM for storing variables and data and 512KB of flash memory for storing sketches. The Mega 2560 Rev 3 however has the most EEPROM which is used for storing variables and data that should be saved when the Arduino loses power.
I’ve found the MKR WiFi 1010 to be a good option if looking for an Arduino with a lot of memory. Its 32 kilobytes of SRAM is decent compared to most other Arduino models. It’s only downside is that you may need to use an external interface (like an SD card) to store data that needs to be protected when the Arduino loses power.
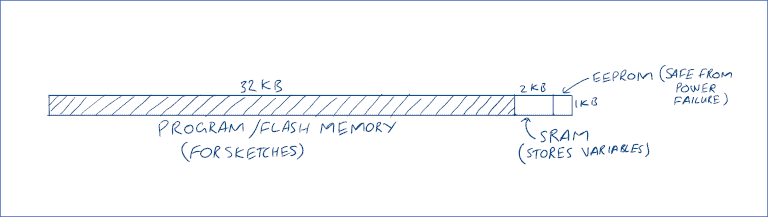
If you’re interested in how the costs of these Arduino models compares, check out the guide I wrote here: chipwired.com/arduino-types-compared/
How much data can be stored on an Arduino
An Arduino can typically store the following amounts of data:
Memory Type | Size | Amount of Data |
---|---|---|
SRAM | 2KB | 1000 numbers |
EEPROM | 1KB | 500 numbers |
Program / Flash | 32KB | 100-1000 lines of code |
Arduino listed prior with a greater memory size can store more data in each of these types of memory. To increase the number of measurements that an Arduino can store, consider using an SD card or uploading data to the cloud. I’ve included more detail about using an SD card further down in this guide. The amount of storage space for a sketch depends on how many libraries are included and how variables and constants are declared in the sketch.
The numbers in this table are based on the Arduino using 2 bytes of memory for each integer (declared using the int
keyword). It is possible to store more numbers in the Arduino memory using the byte
keyword as it only uses 1 byte of memory for each number. An integer can store numbers up to about 64,000, while a byte can only store numbers up to 255.
From what I’ve seen, there is some overhead from a sketch that’s stored in the SRAM. This is related to variables that you declare in your sketch.
How big can an Arduino program be? An Arduino can store around 30,000 instructions in 32 kilobytes of program memory. This can be equivalent to a few hundred lines of code in a sketch, depending on which libraries are used. Some memory may also be reserved for the bootloader or processor functions. It is not possible to add program memory to most Arduino.
Types of Arduino Memory
Data is typically stored on an Arduino using the EEPROM or SRAM memory. Any data stored in SRAM will be lost if the Arduino loses power; EEPROM and flash memory are preserved.
Flash memory is used to store sketches, it can also be referred to as program memory. A computer or external programmer connected to the Arduino can be used to overwrite the flash memory. This is what happens when a sketch is uploaded to the Arduino.
Memory Type | Uses |
---|---|
Flash or Program Memory | Stores the sketch/code. Not lost when the Arduino loses power. |
SRAM – Static Random-Access Memory | Stores current data used by a sketch, data is lost when power is lost. |
EEPROM – Electronically Erasable Programmable Read-Only Memory | To save data in the event the Arduino loses power. |
Memory typically has a fixed lifespan:
- SRAM is expected to last for 20 years
- EEPROM can be written approximately 100,000 times per address
- Flash memory can be programmed approximately 100,000 times
According to research, the SRAM used in an Arduino can last over 20 years. Heavy use of the SRAM may decrease this lifespan by 2-5 years. SRAM typically degrades by block, on an Arduino this may mean the entire SRAM stops working after this number of years. I’ve included a link to the paper in the References section at the end of this guide.
Once the flash or EEPROM memory reache end-of-life they will no longer be able to be programmed. Reading it will read the last data that was programmed into it, or nothing (I haven’t reliably determined which way this happens yet).
How to add memory to an Arduino
Additional memory can be added to an Arduino by using the SPI interface to connect an SD card or other storage device. It is not possible to add RAM directly to an Arduino as there is no bus or support for general purpose memory chips.
To add additional memory to an Arduino using an SD card:
- Get SD card shield
- Connect to SPI
- Include
SPI.h
andSD.h
libraries - Initialise the SD card in the
setup()
function - Open a file to use as memory using
SD.open()
- Read or write to the file using
read()
orprintln()
respectively - Close the file with
close()
when finished.
Some Arduino models include a built-in SD card slot. Currently this only includes the Yun Rev 2 and the MKR Zero. Some of the Portenta models appear to have the ability to add an SD card expansion (I haven’t tried a Portenta board yet), or you can use the SPI interface described above.
The official Arduino Mem shield (designed for MKR) also contains on-board flash memory that can be used in addition to the option to insert an SD card.
What happens when an Arduino runs out of memory
In my guide on how Arduino can crash or hang, I tried to crash an Arduino by making it run out of memory. It was surprisingly robust.
If an Arduino runs out of program memory, the IDE will warn you before uploading. You will not be able to upload a sketch larger than the amount of flash memory available for programs. Try reducing the amount of included libraries or the size of variables to reduce the size of the sketch.
If an Arduino runs out of SRAM due to function calls, it will reset. This typically happens if you call a function from within itself without having a condition to branch somewhere else. If an Arduino runs out of SRAM due to use of malloc()
it will stop allocating you more memory and you can overwrite previously allocated memory.
If an Arduino runs out of EEPROM, nothing will happen, a sketch will overwrite whatever was stored at that address previously. To add EEPROM and save data in the event of power-loss, consider using an SD card or external flash memory. If you have an MKR, the Arduino MKR flash memory shield gives you both SD card and flash memory on one shield, check it out here.
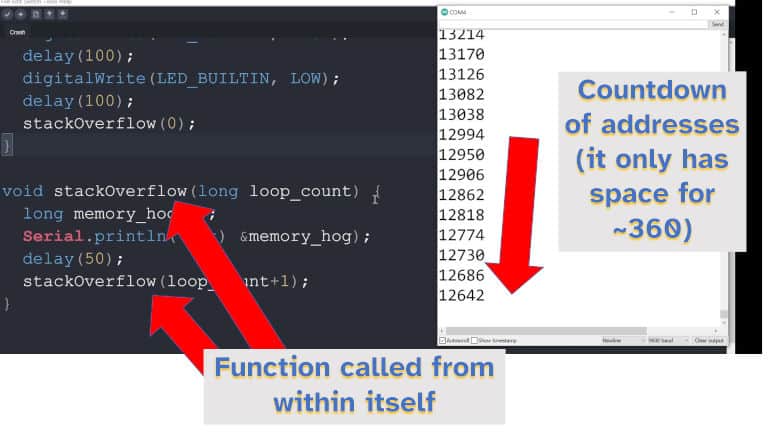
If you’re interested in other ways an Arduino can crash or hang (and how to fix it), check out my guide here: chipwired.com/arduino-crash-hang-guide/
References
- Size of integer used by Arduino
- Lifespan of SRAM is complicated, check out this paper for more details
Chris has a great memory for electronics ;). He’s been experimenting with memory and embedded software for years.